トリガーイベントとは
ある条件に従ってモーションを実行させる機能として「イベント」がありますが、その判定条件を7個まで拡張できる機能が「トリガーイベント」になります。 大まかな動きは、イベント単位では判定条件の成立でフラグを立ていきます。そしてイベントが立てたフラグがすべてTrueになった時にモーションを開始させるものになります。
【サンプル】2つの軸を移動させ、停止間際に別の軸を移動させるモーション
先行で軸0と軸1の移動を開始します。 先行軸の停止間際に、軸2のモーションを開始させる動作が実現できます。
シミュレーション結果
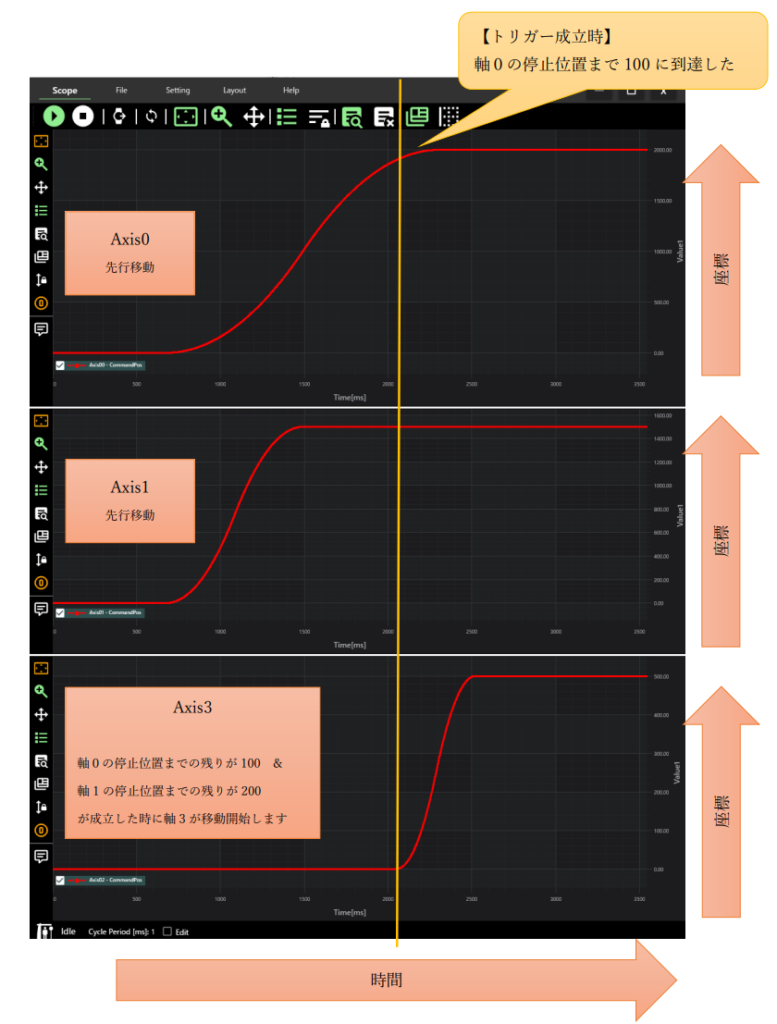
C#コードのサンプル
※WMX3.6で検証したコード
private void Moveトリガーイベント()
{
var CMotion = new CoreMotion(API);
//軸0に対して通常のモーション指令を実行する
var pos = new Motion.PosCommand();
pos.Axis = 0; // 制御軸
pos.Profile.Type = ProfileType.Trapezoidal;
pos.Profile.Velocity = 7000;
pos.Profile.Acc = 3000;
pos.Profile.Dec = 3000;
pos.Target = 2000; // 目的座標
var IsErr = CMotion.Motion.StartMov(pos); // 移動開始
//軸1に対して通常のモーション指令を実行する
pos.Axis = 1; // 制御軸
pos.Profile.Type = ProfileType.Trapezoidal;
pos.Profile.Velocity = 4000;
pos.Profile.Acc = 9000;
pos.Profile.Dec = 9000;
pos.Target = 1500; // 目的座標
IsErr = CMotion.Motion.StartMov(pos); // 移動開始
// ↓この時点で軸0と1は動作しています
// トリガー条件
TriggerEvents tEvent = new TriggerEvents();
tEvent.NumEvents = 2+1; // 登録したいトリガーの個数(条件判定 + フラグ判定)
// トリガー条件1の設定(監視条件)
tEvent.Event[0].InputFunction = TriggerEventInputFunction.DistanceToTarget; // モーションの残り距離を判定
tEvent.Event[0].Input_DistanceToTarget.Axis = 0; // 監視する軸
tEvent.Event[0].Input_DistanceToTarget.Distance = 100; // 監視する残り距離
tEvent.Event[0].OutputFunction = TriggerEventOutputFunction.SetReg;
tEvent.Event[0].Output_SetReg.RegNumber = 0; // 結果判定用レジスタの登録番号
// トリガー条件2の設定(監視条件)
tEvent.Event[1].InputFunction = TriggerEventInputFunction.DistanceToTarget; // モーションの残り距離を判定
tEvent.Event[1].Input_DistanceToTarget.Axis = 1; // 監視する軸
tEvent.Event[1].Input_DistanceToTarget.Distance = 200; // 監視する残り距離
tEvent.Event[1].OutputFunction = TriggerEventOutputFunction.SetReg;
tEvent.Event[1].Output_SetReg.RegNumber = 1; // 結果判定用レジスタの登録番号
//フラグ判定:レジスタ0とレジスタ1の両方がセットされているときにトリガーモーションを開始する
tEvent.Event[2].InputFunction = TriggerEventInputFunction.AndReg; // モーションの残り距離を判定
tEvent.Event[2].Input_AndReg.RegNumber[0] = 0; // 監視するフラグ番号
tEvent.Event[2].Input_AndReg.RegNumber[1] = 1; // 監視するフラグ番号
tEvent.Event[2].OutputFunction = TriggerEventOutputFunction.TriggerMotion; // モーションの実行
// トリガーイベントでのモーション動作
pos.Axis = 2; // 制御軸
pos.Profile.Type = ProfileType.Trapezoidal;
pos.Profile.Velocity = 4000;
pos.Profile.Acc = 9000;
pos.Profile.Dec = 9000;
pos.Target = 500; // 目的座標
IsErr = CMotion.Motion.StartMov(pos, tEvent); // 移動開始
// 軸0と1の条件が整い次第、軸2が動作を開始します
}
コメント